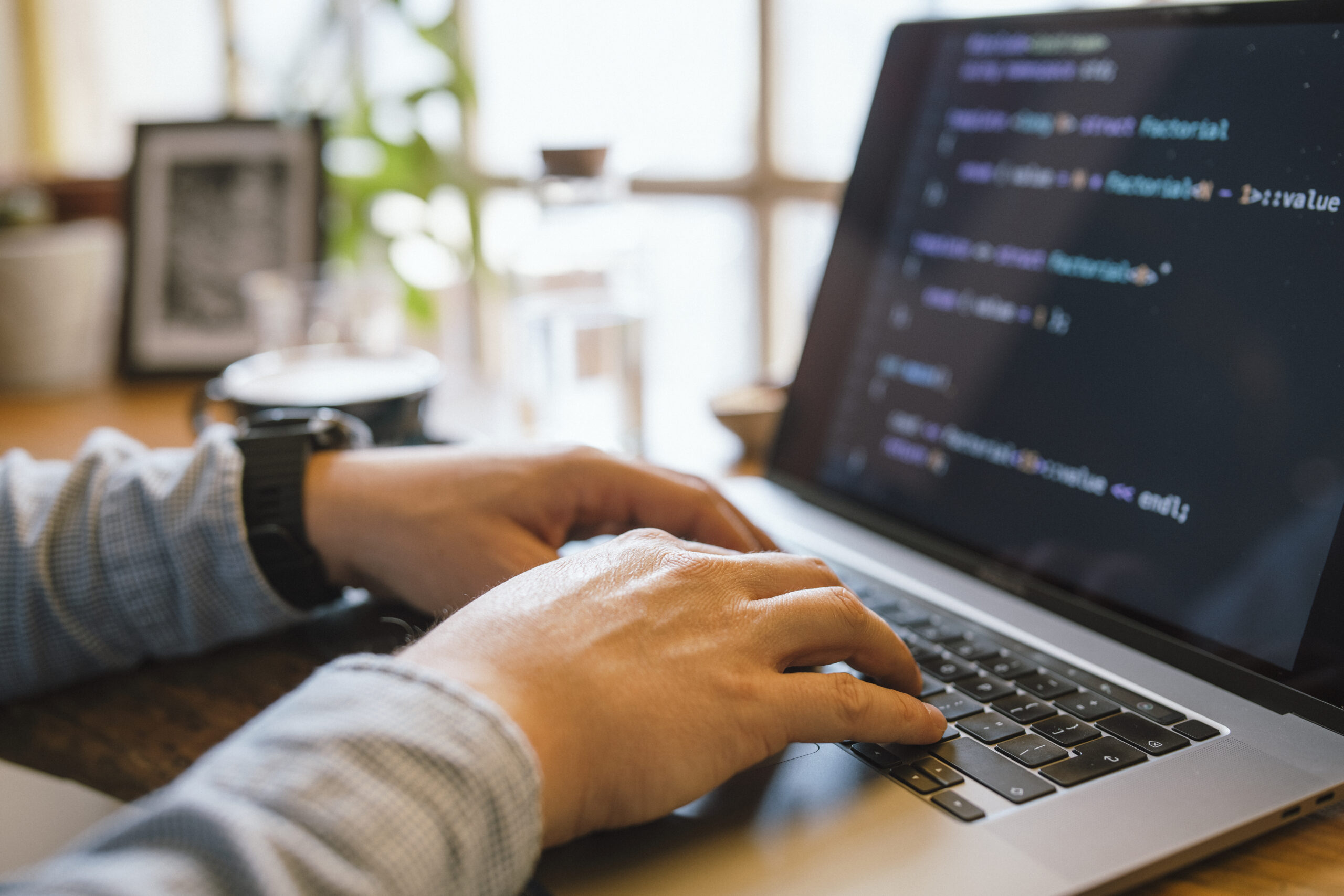
Debugging is One of the more crucial — still normally ignored — expertise in the developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why issues go Incorrect, and Understanding to Consider methodically to resolve troubles proficiently. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially enhance your productivity. Listed here are several strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest strategies builders can elevate their debugging expertise is by mastering the resources they use every day. Though producing code is one particular Portion of improvement, knowing ways to communicate with it efficiently throughout execution is Similarly vital. Present day advancement environments come Outfitted with potent debugging abilities — but a lot of developers only scratch the area of what these equipment can perform.
Just take, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code around the fly. When made use of appropriately, they Permit you to notice just how your code behaves during execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, check out serious-time efficiency metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep Command more than managing procedures and memory administration. Studying these equipment can have a steeper Studying curve but pays off when debugging functionality difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Model Command methods like Git to be familiar with code history, locate the exact minute bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools indicates heading over and above default settings and shortcuts — it’s about building an intimate understanding of your advancement setting making sure that when difficulties crop up, you’re not missing at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often missed — ways in productive debugging is reproducing the situation. In advance of jumping in to the code or creating guesses, builders will need to make a constant environment or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug gets a recreation of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as possible. Check with queries like: What steps brought about the issue? Which ecosystem was it in — growth, staging, or output? Are there any logs, screenshots, or error messages? The more element you might have, the simpler it results in being to isolate the exact disorders beneath which the bug occurs.
As soon as you’ve collected ample info, endeavor to recreate the trouble in your neighborhood atmosphere. This may imply inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at creating automatic checks that replicate the edge situations or point out transitions involved. These assessments don't just aid expose the situation but also avert regressions Down the road.
Occasionally, The problem may very well be atmosphere-distinct — it'd happen only on specific running devices, browsers, or under certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these bugs.
Reproducing the problem isn’t just a stage — it’s a frame of mind. It calls for endurance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you happen to be by now midway to correcting it. That has a reproducible state of affairs, You may use your debugging tools more successfully, check possible fixes safely, and communicate additional Plainly with the staff or people. It turns an summary grievance into a concrete challenge — and that’s where by builders prosper.
Read through and Recognize the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Erroneous. In lieu of observing them as aggravating interruptions, developers should master to take care of error messages as direct communications within the process. They typically let you know precisely what transpired, wherever it took place, and occasionally even why it transpired — if you know the way to interpret them.
Commence by studying the information meticulously and in comprehensive. A lot of developers, specially when below time tension, glance at the very first line and straight away start off creating assumptions. But further inside the mistake stack or logs may possibly lie the accurate root bring about. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it position to a specific file and line variety? What module or function activated it? These questions can information your investigation and point you toward the liable code.
It’s also practical to grasp the terminology of your programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable patterns, and Mastering to recognize these can dramatically hasten your debugging process.
Some problems are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake happened. Examine relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a additional economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic info during development, Facts for typical gatherings (like profitable start off-ups), WARN for potential challenges that don’t split the appliance, ERROR for precise troubles, and Deadly when the procedure can’t continue on.
Stay away from flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on critical activities, state improvements, input/output values, and important determination points in the code.
Format your log messages Plainly and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. By using a well-imagined-out logging tactic, you are able to decrease the time it's going to take to spot concerns, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Feel Similar to a Detective
Debugging is not merely a technical activity—it is a method of investigation. To successfully recognize and deal with bugs, builders must method the method just like a detective fixing a secret. This mentality assists break down sophisticated troubles into workable sections and abide by clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or general performance challenges. The same as a detective surveys a crime scene, collect as much related information as you can with out jumping to conclusions. Use logs, test instances, and user reviews to piece with each other a clear image of what’s happening.
Subsequent, type hypotheses. Inquire your self: What might be creating this conduct? Have any modifications not too long ago been created for the codebase? Has this problem occurred before less than identical situation? The purpose is always to narrow down alternatives and establish likely culprits.
Then, check your theories systematically. Try to recreate the condition in the controlled ecosystem. In case you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, inquire your code questions and Permit the outcomes guide you closer to the reality.
Shell out close notice to tiny particulars. Bugs normally conceal in the minimum expected spots—like a lacking semicolon, an off-by-one mistake, or a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may perhaps hide the true problem, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future issues and support others realize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more effective at uncovering hidden troubles in elaborate systems.
Publish Assessments
Writing exams is one of the best tips on how to improve your debugging competencies and overall advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self-assurance when generating improvements towards your codebase. A perfectly-analyzed software is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when a difficulty happens.
Begin with unit exams, which center on unique functions or modules. These compact, isolated tests can quickly reveal whether a specific bit of logic is Doing the job as envisioned. When a exam fails, you straight away know wherever to glance, drastically minimizing time invested debugging. Unit checks are In particular handy for catching regression bugs—troubles that reappear right after previously remaining preset.
Following, integrate integration tests and close-to-conclude exams into your workflow. These assist ensure that many areas of your application do the job jointly easily. They’re especially practical for catching bugs that arise in complicated units with many elements or products and services interacting. If anything breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a feature adequately, you will need to be familiar with its inputs, anticipated outputs, and edge cases. This amount of understanding Obviously prospects to raised code structure and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a powerful initial step. As soon as the check fails continuously, you are able to center on correcting the bug and observe your take a look at go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the disheartening guessing game into a structured and predictable process—aiding you catch additional bugs, a lot quicker and much more reliably.
Just take Breaks
When debugging a difficult challenge, it’s easy to become immersed in the issue—watching your display screen for several hours, seeking solution following Answer. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your intellect, cut down frustration, and often see The difficulty from the new standpoint.
If you're much too near the code for far too extensive, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just hrs previously. On this state, your brain becomes fewer economical at challenge-fixing. A short wander, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your emphasis. Several developers report getting the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, nonetheless it basically results in a lot quicker and more effective debugging In the long term.
In brief, getting breaks is not a sign of weak point—it’s a sensible method. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It is really an opportunity to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something useful for those who make an effort to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of crucial queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or being familiar with and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you acquired. Eventually, you’ll begin to see designs—recurring problems or common mistakes—you could proactively prevent.
In crew environments, sharing Everything you've discovered from the bug with the peers can be Primarily highly effective. No matter whether it’s through a Slack information, a short create-up, or A fast expertise-sharing session, assisting Many others stay away from get more info the exact same difficulty boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. In the end, many of the greatest builders aren't those who create great code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll arrive absent a smarter, more capable developer thanks to it.
Conclusion
Bettering your debugging competencies requires time, follow, and tolerance — however the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep within a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.